Jquery One Action on Click Then Another on Click Again
Chapter 15Handling Events
You have power over your listen—not exterior events. Realize this, and you will find force.
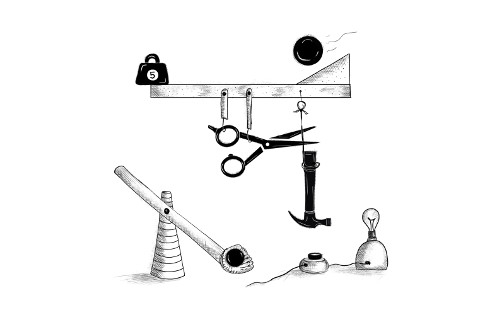
Some programs work with directly user input, such as mouse and keyboard actions. That kind of input isn't available as a well-organized data structure—it comes in piece by slice, in existent fourth dimension, and the program is expected to respond to information technology as information technology happens.
Upshot handlers
Imagine an interface where the only manner to find out whether a key on the keyboard is existence pressed is to read the current country of that cardinal. To be able to react to keypresses, you would have to constantly read the key'due south state so that you'd catch information technology before information technology's released once again. It would be dangerous to perform other time-intensive computations since you might miss a keypress.
Some archaic machines exercise handle input like that. A pace upward from this would be for the hardware or operating organization to notice the keypress and put information technology in a queue. A program can and then periodically check the queue for new events and react to what it finds in that location.
Of grade, it has to think to expect at the queue, and to do information technology often, because any time between the key beingness pressed and the program noticing the effect will cause the software to experience unresponsive. This approach is called polling. Most programmers prefer to avert it.
A better mechanism is for the system to actively notify our code when an event occurs. Browsers do this past allowing us to annals functions as handlers for specific events.
< p >Click this document to activate the handler.</ p > < script > window.addEventListener("click", () => { panel.log("You knocked?"); }); </ script >
The window
binding refers to a congenital-in object provided by the browser. It represents the browser window that contains the document. Calling its addEventListener
method registers the second argument to be called whenever the outcome described by its first statement occurs.
Events and DOM nodes
Each browser event handler is registered in a context. In the previous instance we chosen addEventListener
on the window
object to register a handler for the whole window. Such a method can likewise exist found on DOM elements and some other types of objects. Event listeners are called only when the event happens in the context of the object they are registered on.
< button >Click me</ button > < p >No handler here.</ p > < script > let button = document.querySelector("button"); button.addEventListener("click", () => { console.log("Button clicked."); }); </ script >
That example attaches a handler to the button node. Clicks on the button cause that handler to run, but clicks on the remainder of the document do not.
Giving a node an onclick
attribute has a like effect. This works for most types of events—you can attach a handler through the aspect whose proper noun is the event name with on
in front of it.
But a node tin have only ane onclick
attribute, so you can annals but one handler per node that way. The addEventListener
method allows yous to add any number of handlers so that information technology is safe to add handlers even if there is already another handler on the element.
The removeEventListener
method, called with arguments similar to addEventListener
, removes a handler.
< push >Act-once button</ button > < script > let button = document.querySelector("button"); office once() { console.log("Washed."); button.removeEventListener("click", once); } push button.addEventListener("click", once); </ script >
The function given to removeEventListener
has to be the same function value that was given to addEventListener
. Then, to unregister a handler, y'all'll want to give the function a name (in one case
, in the example) to be able to pass the same part value to both methods.
Consequence objects
Though nosotros have ignored information technology so far, effect handler functions are passed an argument: the event object. This object holds additional information about the event. For example, if we desire to know which mouse push was pressed, we can look at the event object's push button
property.
< button >Click me any way you want</ push > < script > let button = certificate.querySelector("push"); push button.addEventListener("mousedown", event => { if (event.push button == 0) { console.log("Left button"); } else if (event.button == 1) { panel.log("Middle button"); } else if (event.push == 2) { panel.log("Correct button"); } }); </ script >
The information stored in an event object differs per type of event. Nosotros'll discuss dissimilar types afterward in the chapter. The object's type
property ever holds a string identifying the event (such equally "click"
or "mousedown"
).
Propagation
For most upshot types, handlers registered on nodes with children will likewise receive events that happen in the children. If a button inside a paragraph is clicked, event handlers on the paragraph volition besides see the click result.
But if both the paragraph and the button have a handler, the more specific handler—the one on the push button—gets to go kickoff. The event is said to propagate outward, from the node where it happened to that node's parent node and on to the root of the document. Finally, after all handlers registered on a specific node have had their plough, handlers registered on the whole window get a adventure to respond to the result.
At any point, an outcome handler tin call the stopPropagation
method on the event object to foreclose handlers further up from receiving the event. This tin can be useful when, for example, you have a button inside another clickable element and you don't want clicks on the button to activate the outer element's click behavior.
The following case registers "mousedown"
handlers on both a button and the paragraph around it. When clicked with the correct mouse button, the handler for the push calls stopPropagation
, which will forestall the handler on the paragraph from running. When the button is clicked with some other mouse button, both handlers will run.
< p >A paragraph with a < button >push</ button >.</ p > < script > let para = certificate.querySelector("p"); allow push = document.querySelector("push button"); para.addEventListener("mousedown", () => { console.log("Handler for paragraph."); }); push.addEventListener("mousedown", event => { console.log("Handler for push."); if (issue.push == ii) effect.stopPropagation(); }); </ script >
Most upshot objects have a target
property that refers to the node where they originated. You can use this property to ensure that you're not accidentally handling something that propagated upwards from a node you do not want to handle.
It is besides possible to use the target
belongings to cast a broad internet for a specific blazon of consequence. For example, if y'all have a node containing a long list of buttons, information technology may exist more user-friendly to register a single click handler on the outer node and have information technology use the target
property to figure out whether a push button was clicked, rather than annals private handlers on all of the buttons.
< push >A</ button > < button >B</ button > < button >C</ button > < script > document.torso.addEventListener("click", event => { if (event.target.nodeName == "Push button") { console.log("Clicked", result.target.textContent); } }); </ script >
Default actions
Many events have a default action associated with them. If y'all click a link, y'all volition exist taken to the link's target. If you lot press the downward arrow, the browser volition roll the page down. If you right-click, you'll get a context menu. And and then on.
For nearly types of events, the JavaScript event handlers are called before the default beliefs takes identify. If the handler doesn't want this normal behavior to happen, typically because it has already taken care of handling the event, it tin can call the preventDefault
method on the consequence object.
This can be used to implement your own keyboard shortcuts or context menu. It can as well be used to obnoxiously interfere with the behavior that users expect. For instance, hither is a link that cannot be followed:
< a href="https://developer.mozilla.org/" >MDN</ a > < script > allow link = certificate.querySelector("a"); link.addEventListener("click", effect => { console.log("Nope."); event.preventDefault(); }); </ script >
Try not to practise such things unless you have a really good reason to. It'll be unpleasant for people who utilize your page when expected beliefs is broken.
Depending on the browser, some events can't be intercepted at all. On Chrome, for instance, the keyboard shortcut to close the electric current tab (control-W or control-Westward) cannot be handled past JavaScript.
Key events
When a key on the keyboard is pressed, your browser fires a "keydown"
issue. When information technology is released, you become a "keyup"
issue.
< p >This page turns violet when you agree the V key.</ p > < script > window.addEventListener("keydown", upshot => { if (event.key == "v") { document.body.manner.background = "violet"; } }); window.addEventListener("keyup", event => { if (event.central == "v") { certificate.trunk.style.background = ""; } }); </ script >
Despite its proper noun, "keydown"
fires not simply when the cardinal is physically pushed downwards. When a primal is pressed and held, the event fires once again every time the key repeats. Sometimes you have to exist careful about this. For example, if you add together a button to the DOM when a primal is pressed and remove it over again when the key is released, you might accidentally add hundreds of buttons when the fundamental is held downward longer.
The example looked at the cardinal
holding of the outcome object to run into which cardinal the effect is virtually. This property holds a string that, for near keys, corresponds to the thing that pressing that key would blazon. For special keys such as enter, it holds a string that names the central ("Enter"
, in this case). If y'all concur shift while pressing a key, that might also influence the name of the primal—"v"
becomes "V"
, and "1"
may get "!"
, if that is what pressing shift-1 produces on your keyboard.
Modifier keys such every bit shift, control, alt, and meta (command on Mac) generate central events only like normal keys. Simply when looking for key combinations, you can likewise observe out whether these keys are held downwards by looking at the shiftKey
, ctrlKey
, altKey
, and metaKey
properties of keyboard and mouse events.
< p >Printing Command-Space to continue.</ p > < script > window.addEventListener("keydown", consequence => { if (event.cardinal == " " & & event.ctrlKey) { console.log("Continuing!"); } }); </ script >
The DOM node where a key result originates depends on the chemical element that has focus when the central is pressed. Near nodes cannot take focus unless yous give them a tabindex
aspect, merely things like links, buttons, and form fields tin. Nosotros'll come up dorsum to form fields in Chapter eighteen. When zip in particular has focus, document.body
acts as the target node of primal events.
When the user is typing text, using key events to figure out what is being typed is problematic. Some platforms, near notably the virtual keyboard on Android phones, don't fire fundamental events. But even when you have an old-fashioned keyboard, some types of text input don't friction match key presses in a straightforward way, such as input method editor (IME) software used by people whose scripts don't fit on a keyboard, where multiple key strokes are combined to create characters.
To find when something was typed, elements that you can type into, such as the <input>
and <textarea>
tags, fire "input"
events whenever the user changes their content. To get the actual content that was typed, it is best to direct read it from the focused field. Chapter 18 will testify how.
Arrow events
In that location are currently two widely used ways to bespeak at things on a screen: mice (including devices that act similar mice, such as touchpads and trackballs) and touchscreens. These produce different kinds of events.
Mouse clicks
Pressing a mouse button causes a number of events to burn down. The "mousedown"
and "mouseup"
events are similar to "keydown"
and "keyup"
and fire when the button is pressed and released. These happen on the DOM nodes that are immediately beneath the mouse pointer when the event occurs.
After the "mouseup"
result, a "click"
event fires on the near specific node that contained both the press and the release of the push button. For example, if I press down the mouse push button on one paragraph and so motility the pointer to another paragraph and release the button, the "click"
event will happen on the chemical element that contains both those paragraphs.
If two clicks happen close together, a "dblclick"
(double-click) event as well fires, subsequently the 2nd click event.
To get precise information about the place where a mouse upshot happened, yous can look at its clientX
and clientY
backdrop, which contain the issue's coordinates (in pixels) relative to the top-left corner of the window, or pageX
and pageY
, which are relative to the acme-left corner of the whole document (which may be dissimilar when the window has been scrolled).
The following implements a primitive drawing program. Every fourth dimension y'all click the document, it adds a dot under your mouse pointer. See Chapter 19 for a less archaic drawing program.
< style > body { pinnacle: 200px; background: beige; } .dot { height: 8px; width: 8px; border-radius: 4px; background: blue; position: absolute; } </ way > < script > window.addEventListener("click", result => { let dot = document.createElement("div"); dot.className = "dot"; dot.style.left = (event.pageX - 4) + "px"; dot.style.top = (event.pageY - 4) + "px"; document.trunk.appendChild(dot); }); </ script >
Mouse motion
Every time the mouse pointer moves, a "mousemove"
event is fired. This outcome can exist used to rail the position of the mouse. A common state of affairs in which this is useful is when implementing some form of mouse-dragging functionality.
As an instance, the post-obit program displays a bar and sets upwards event handlers so that dragging to the left or correct on this bar makes it narrower or wider:
< p >Elevate the bar to change its width:</ p > < div style="background: orange; width: 60px; peak: 20px" > </ div > < script > permit lastX; permit bar = certificate.querySelector("div"); bar.addEventListener("mousedown", event => { if (event.push == 0) { lastX = event.clientX; window.addEventListener("mousemove", moved); event.preventDefault(); } }); function moved(event) { if (event.buttons == 0) { window.removeEventListener("mousemove", moved); } else { let dist = issue.clientX - lastX; let newWidth = Math.max(x, bar.offsetWidth + dist); bar.style.width = newWidth + "px"; lastX = outcome.clientX; } } </ script >
Note that the "mousemove"
handler is registered on the whole window. Even if the mouse goes exterior of the bar during resizing, as long as the button is held we however want to update its size.
We must finish resizing the bar when the mouse push button is released. For that, we can utilize the buttons
belongings (notation the plural), which tells us well-nigh the buttons that are currently held down. When this is zero, no buttons are down. When buttons are held, its value is the sum of the codes for those buttons—the left button has lawmaking 1, the right push button 2, and the eye i 4. With the left and correct buttons held, for example, the value of buttons
will be 3.
Notation that the club of these codes is unlike from the one used past push button
, where the eye button came before the right 1. Every bit mentioned, consistency isn't really a strong bespeak of the browser's programming interface.
Touch events
The style of graphical browser that we use was designed with mouse interfaces in mind, at a time where touchscreens were rare. To make the Web "piece of work" on early on touchscreen phones, browsers for those devices pretended, to a certain extent, that touch events were mouse events. If y'all tap your screen, you'll get "mousedown"
, "mouseup"
, and "click"
events.
Only this illusion isn't very robust. A touchscreen works differently from a mouse: it doesn't have multiple buttons, y'all can't runway the finger when it isn't on the screen (to simulate "mousemove"
), and it allows multiple fingers to exist on the screen at the aforementioned time.
Mouse events encompass affect interaction simply in straightforward cases—if y'all add a "click"
handler to a button, affect users will withal be able to employ it. Simply something like the resizeable bar in the previous example does not work on a touchscreen.
There are specific effect types fired by touch interaction. When a finger starts touching the screen, you get a "touchstart"
consequence. When it is moved while touching, "touchmove"
events fire. Finally, when it stops touching the screen, you'll see a "touchend"
effect.
Considering many touchscreens can detect multiple fingers at the same time, these events don't take a single set of coordinates associated with them. Rather, their effect objects take a touches
property, which holds an assortment-similar object of points, each of which has its own clientX
, clientY
, pageX
, and pageY
properties.
You could practise something like this to show blood-red circles around every touching finger:
< style > dot { position: absolute; display: block; border: 2px solid red; border-radius: 50px; acme: 100px; width: 100px; } </ style > < p >Touch this page</ p > < script > role update(result) { for (let dot; dot = certificate.querySelector("dot");) { dot.remove(); } for (allow i = 0; i < outcome.touches.length; i ++) { let {pageX, pageY} = effect.touches[i]; permit dot = document.createElement("dot"); dot.way.left = (pageX - 50) + "px"; dot.style.top = (pageY - l) + "px"; document.body.appendChild(dot); } } window.addEventListener("touchstart", update); window.addEventListener("touchmove", update); window.addEventListener("touchend", update); </ script >
You lot'll oft want to call preventDefault
in bear on effect handlers to override the browser'southward default beliefs (which may include scrolling the folio on swiping) and to prevent the mouse events from beingness fired, for which you may likewise have a handler.
Gyre events
Whenever an chemical element is scrolled, a "coil"
consequence is fired on it. This has diverse uses, such as knowing what the user is currently looking at (for disabling off-screen animations or sending spy reports to your evil headquarters) or showing some indication of progress (by highlighting role of a table of contents or showing a page number).
The following case draws a progress bar above the document and updates it to make full up as y'all curlicue downwards:
< fashion > #progress { border-lesser: 2px solid blue; width: 0; position: fixed; top: 0; left: 0; } </ fashion > < div id="progress" > </ div > < script > document.body.appendChild(document.createTextNode( "supercalifragilisticexpialidocious ".repeat(1000))); let bar = document.querySelector("#progress"); window.addEventListener("scroll", () => { let max = document.torso.scrollHeight - innerHeight; bar.style.width = `${(pageYOffset / max) * 100 } %`; }); </ script >
Giving an element a position
of fixed
acts much like an absolute
position but also prevents it from scrolling along with the residual of the document. The effect is to make our progress bar stay at the height. Its width is changed to signal the current progress. Nosotros utilise %
, rather than px
, as a unit when setting the width so that the element is sized relative to the folio width.
The global innerHeight
binding gives us the elevation of the window, which we have to subtract from the total scrollable peak—you lot can't continue scrolling when you hit the bottom of the document. There's as well an innerWidth
for the window width. By dividing pageYOffset
, the current scroll position, past the maximum scroll position and multiplying by 100, we become the percentage for the progress bar.
Calling preventDefault
on a scroll event does not forestall the scrolling from happening. In fact, the event handler is chosen simply after the scrolling takes place.
Focus events
When an element gains focus, the browser fires a "focus"
event on information technology. When it loses focus, the element gets a "blur"
event.
Unlike the events discussed earlier, these 2 events do not propagate. A handler on a parent chemical element is not notified when a child element gains or loses focus.
The following instance displays help text for the text field that currently has focus:
< p >Name: < input type="text" information-assistance="Your full name" > </ p > < p >Age: < input type="text" data-help="Your age in years" > </ p > < p id="help" > </ p > < script > let help = document.querySelector("#assistance"); allow fields = document.querySelectorAll("input"); for (permit field of Array.from(fields)) { field.addEventListener("focus", event => { permit text = effect.target.getAttribute("data-help"); aid.textContent = text; }); field.addEventListener("blur", effect => { assist.textContent = ""; }); } </ script >
The window object will receive "focus"
and "blur"
events when the user moves from or to the browser tab or window in which the document is shown.
Load event
When a page finishes loading, the "load"
effect fires on the window and the document torso objects. This is often used to schedule initialization actions that crave the whole document to accept been built. Remember that the content of <script>
tags is run immediately when the tag is encountered. This may be also shortly, for example when the script needs to practise something with parts of the document that appear after the <script>
tag.
Elements such as images and script tags that load an external file as well have a "load"
event that indicates the files they reference were loaded. Similar the focus-related events, loading events do not propagate.
When a folio is closed or navigated abroad from (for example, by following a link), a "beforeunload"
consequence fires. The main utilise of this result is to prevent the user from accidentally losing work past closing a certificate. If y'all preclude the default beliefs on this outcome and set the returnValue
property on the event object to a string, the browser volition testify the user a dialog request if they actually desire to leave the page. That dialog might include your cord, but because some malicious sites attempt to use these dialogs to confuse people into staying on their page to look at dodgy weight loss ads, most browsers no longer brandish them.
Events and the consequence loop
In the context of the outcome loop, as discussed in Chapter 11, browser event handlers behave like other asynchronous notifications. They are scheduled when the event occurs only must wait for other scripts that are running to terminate before they get a chance to run.
The fact that events tin can exist processed only when zilch else is running means that, if the outcome loop is tied upward with other work, any interaction with the page (which happens through events) volition be delayed until at that place'south time to process it. So if you schedule too much work, either with long-running event handlers or with lots of curt-running ones, the page will go tedious and cumbersome to employ.
For cases where you really do want to do some time-consuming thing in the background without freezing the page, browsers provide something called spider web workers. A worker is a JavaScript process that runs alongside the main script, on its own timeline.
Imagine that squaring a number is a heavy, long-running computation that nosotros want to perform in a separate thread. Nosotros could write a file chosen code/
that responds to messages by computing a square and sending a message back.
addEventListener("message", event => { postMessage(issue.information * issue.data); });
To avoid the problems of having multiple threads touching the same data, workers do non share their global telescopic or any other data with the chief script'due south environment. Instead, you take to communicate with them past sending messages dorsum and forth.
This code spawns a worker running that script, sends it a few messages, and outputs the responses.
allow squareWorker = new Worker("code/squareworker.js"); squareWorker.addEventListener("message", event => { console.log("The worker responded:", event.data); }); squareWorker.postMessage(10); squareWorker.postMessage(24);
The postMessage
function sends a message, which will crusade a "message"
outcome to fire in the receiver. The script that created the worker sends and receives messages through the Worker
object, whereas the worker talks to the script that created it by sending and listening directly on its global telescopic. Only values that tin can exist represented every bit JSON tin can exist sent equally letters—the other side will receive a copy of them, rather than the value itself.
Timers
We saw the setTimeout
function in Chapter 11. It schedules another function to exist chosen subsequently, after a given number of milliseconds.
Sometimes yous need to cancel a function you take scheduled. This is washed by storing the value returned past setTimeout
and calling clearTimeout
on it.
let bombTimer = setTimeout(() => { panel.log("Smash!"); }, 500); if (Math.random() < 0.v) { console.log("Defused."); clearTimeout(bombTimer); }
The cancelAnimationFrame
function works in the same mode as clearTimeout
—calling it on a value returned by requestAnimationFrame
will cancel that frame (assuming it hasn't already been called).
A similar ready of functions, setInterval
and clearInterval
, are used to ready timers that should repeat every X milliseconds.
let ticks = 0; let clock = setInterval(() => { console.log("tick", ticks ++); if (ticks == 10) { clearInterval(clock); console.log("finish."); } }, 200);
Debouncing
Some types of events have the potential to fire chop-chop, many times in a row (the "mousemove"
and "gyre"
events, for case). When handling such events, you lot must be conscientious not to do anything too time-consuming or your handler will accept upwards and then much time that interaction with the certificate starts to feel tiresome.
If you do need to do something nontrivial in such a handler, y'all can utilize setTimeout
to brand sure you are not doing information technology likewise often. This is usually called debouncing the upshot. There are several slightly different approaches to this.
In the beginning example, we want to react when the user has typed something, only we don't desire to do it immediately for every input upshot. When they are typing quickly, we simply desire to look until a suspension occurs. Instead of immediately performing an action in the upshot handler, we set a timeout. We as well clear the previous timeout (if whatever) then that when events occur close together (closer than our timeout delay), the timeout from the previous issue volition be canceled.
< textarea >Type something here...</ textarea > < script > let textarea = document.querySelector("textarea"); let timeout; textarea.addEventListener("input", () => { clearTimeout(timeout); timeout = setTimeout(() => console.log("Typed!"), 500); }); </ script >
Giving an undefined value to clearTimeout
or calling it on a timeout that has already fired has no effect. Thus, we don't have to be careful about when to call it, and we but exercise so for every result.
We tin use a slightly unlike design if we want to space responses and then that they're separated by at least a sure length of time but want to fire them during a series of events, not just afterward. For example, we might desire to respond to "mousemove"
events by showing the electric current coordinates of the mouse only only every 250 milliseconds.
< script > let scheduled = zero; window.addEventListener("mousemove", event => { if (! scheduled) { setTimeout(() => { document.body.textContent = `Mouse at ${ scheduled.pageX } , ${ scheduled.pageY } `; scheduled = null; }, 250); } scheduled = issue; }); </ script >
Summary
Result handlers go far possible to detect and react to events happening in our spider web folio. The addEventListener
method is used to register such a handler.
Each event has a type ("keydown"
, "focus"
, and so on) that identifies information technology. Nigh events are chosen on a specific DOM element and then propagate to that chemical element's ancestors, allowing handlers associated with those elements to handle them.
When an outcome handler is chosen, it is passed an event object with additional data nearly the effect. This object also has methods that allow us to cease further propagation (stopPropagation
) and forestall the browser'due south default treatment of the event (preventDefault
).
Pressing a fundamental fires "keydown"
and "keyup"
events. Pressing a mouse push fires "mousedown"
, "mouseup"
, and "click"
events. Moving the mouse fires "mousemove"
events. Touchscreen interaction will issue in "touchstart"
, "touchmove"
, and "touchend"
events.
Scrolling can exist detected with the "ringlet"
issue, and focus changes can exist detected with the "focus"
and "mistiness"
events. When the certificate finishes loading, a "load"
event fires on the window.
Exercises
Balloon
Write a page that displays a balloon (using the balloon emoji, 🎈). When y'all press the up arrow, information technology should inflate (grow) 10 percent, and when you press the down pointer, it should deflate (shrink) 10 pct.
You lot can control the size of text (emoji are text) past setting the font-size
CSS belongings (style.fontSize
) on its parent element. Remember to include a unit in the value—for example, pixels (10px
).
The cardinal names of the arrow keys are "ArrowUp"
and "ArrowDown"
. Make certain the keys change only the balloon, without scrolling the page.
When that works, add a feature where, if you blow upwardly the balloon by a certain size, it explodes. In this example, exploding means that it is replaced with an 💥 emoji, and the event handler is removed (so that you can't inflate or deflate the explosion).
< p >🎈</ p > < script > </ script >
You'll desire to register a handler for the "keydown"
upshot and look at upshot.primal
to figure out whether the upwards or down pointer central was pressed.
The current size can be kept in a binding and then that you tin base the new size on it. It'll be helpful to define a function that updates the size—both the binding and the style of the airship in the DOM—so that you tin call information technology from your result handler, and possibly also once when starting, to set the initial size.
You can change the balloon to an explosion by replacing the text node with another one (using replaceChild
) or by setting the textContent
property of its parent node to a new cord.
Mouse trail
In JavaScript's early on days, which was the loftier time of gaudy home pages with lots of animated images, people came upward with some truly inspiring ways to use the linguistic communication.
One of these was the mouse trail—a series of elements that would follow the mouse arrow as you lot moved it across the page.
In this exercise, I desire you to implement a mouse trail. Apply admittedly positioned <div>
elements with a stock-still size and background color (refer to the code in the "Mouse Clicks" section for an example). Create a bunch of such elements and, when the mouse moves, brandish them in the wake of the mouse pointer.
At that place are various possible approaches hither. You lot can make your solution as unproblematic or as complex as yous want. A unproblematic solution to starting time with is to go along a fixed number of trail elements and cycle through them, moving the next one to the mouse'due south current position every fourth dimension a "mousemove"
event occurs.
< mode > .trail { position: absolute; summit: 6px; width: 6px; border-radius: 3px; background: teal; } body { pinnacle: 300px; } </ way > < script > </ script >
Creating the elements is all-time done with a loop. Append them to the document to make them evidence up. To be able to access them later to change their position, you'll want to store the elements in an array.
Cycling through them tin exist washed by keeping a counter variable and adding i to information technology every fourth dimension the "mousemove"
outcome fires. The residue operator (% elements.
) can and so be used to get a valid array alphabetize to selection the chemical element you want to position during a given issue.
Some other interesting event can be achieved past modeling a unproblematic physics system. Utilize the "mousemove"
event only to update a pair of bindings that track the mouse position. And so use requestAnimationFrame
to simulate the trailing elements being attracted to the position of the mouse pointer. At every animation stride, update their position based on their position relative to the pointer (and, optionally, a speed that is stored for each chemical element). Figuring out a proficient style to practise this is up to you.
Tabs
Tabbed panels are widely used in user interfaces. They allow yous to select an interface panel past choosing from a number of tabs "sticking out" above an element.
In this do you lot must implement a simple tabbed interface. Write a function, asTabs
, that takes a DOM node and creates a tabbed interface showing the kid elements of that node. It should insert a list of <button>
elements at the acme of the node, i for each kid element, containing text retrieved from the information-tabname
aspect of the kid. All but one of the original children should be hidden (given a display
style of none
). The currently visible node can exist selected by clicking the buttons.
When that works, extend information technology to style the push for the currently selected tab differently then that it is obvious which tab is selected.
< tab-panel > < div data-tabname="ane" >Tab one</ div > < div data-tabname="2" >Tab two</ div > < div data-tabname="iii" >Tab 3</ div > </ tab-panel > < script > function asTabs(node) { } asTabs(document.querySelector("tab-panel")); </ script >
One pitfall you might run into is that you tin can't directly use the node's childNodes
property every bit a collection of tab nodes. For 1 thing, when you add together the buttons, they will also get kid nodes and end up in this object because it is a live data structure. For some other, the text nodes created for the whitespace between the nodes are as well in childNodes
just should non go their ain tabs. You lot tin use children
instead of childNodes
to ignore text nodes.
You could commencement by building upwards an assortment of tabs and so that you have easy access to them. To implement the styling of the buttons, you could store objects that incorporate both the tab panel and its push button.
I recommend writing a separate function for irresolute tabs. Y'all can either shop the previously selected tab and change only the styles needed to hide that and evidence the new one, or y'all can just update the style of all tabs every time a new tab is selected.
You might want to telephone call this function immediately to brand the interface showtime with the first tab visible.
nelsontiolsell1965.blogspot.com
Source: https://eloquentjavascript.net/15_event.html
Post a Comment for "Jquery One Action on Click Then Another on Click Again"